Home / Blog / Insights / Custom jQuery Confirm Dialog in ASP.NET
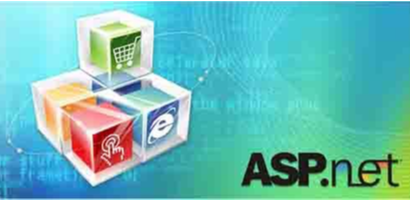
•
Custom jQuery Confirm Dialog in ASP.NET
For one of our new SharePoint modal dialog forms, a button needs to prompt the user whether or not they want to check in a file they are working on. The first solution that comes to mind is JavaScript’s window.confirm
dialog box.
[html light=”true”]
<asp:Button
ID=”btnOk”
runat=”server”
text=”OK”
OnClick=”btnOK_Click”
OnClientClick=”return confirm(‘Do you want to check in?’);”/>
[/html]
The result is a generic dialog that looks different on each browser, does not follow the site’s styling, and has “OK” and “Cancel” buttons when “Yes” and “No” buttons would make more sense. Unfortunately, all of these problems are out of our control.
So what can we do? Make our own using the jQueryUI dialog control!
[html light=”true”]
<asp:Button
ID=”btnOk”
runat=”server”
text=”OK”
OnClick=”btnOk_Click”
OnClientClick=”return confirmCheckIn();”/>
[/html]
[javascript]
function confirmCheckIn() {
jQuery(‘<div>’)
.html(“<p>Do you want to check in?</p>”)
.dialog({
autoOpen: true,
modal: true,
title: “Check in?”,
buttons: {
“No”: function () {
jQuery(this).dialog(“close”);
return false; // don’t do stuff
},
“Yes”: function () {
jQuery(this).dialog(“close”);
return true; // do stuff
}
},
close: function () {
jQuery(this).remove();
return false; // don’t do stuff
}
});
}
[/javascript]
Now we have a dialog that looks the same across all browsers, matches the site’s styling, and has intuitive “Yes” and “No” buttons.
The dialog looks great and all, but it doesn’t work correctly. The button’s back-end click handler does not wait for user input before running because the dialog’s “Yes”, “No”, and “close” handlers are implemented through callbacks, and thus, does not stop the execution of JavaScript to wait for user input (whereas window.confirm
does).
The solution:
[html light=”true”]
<asp:Button
ID=”btnOk”
runat=”server”
text=”OK”
OnClick=”btnOk_Click”
OnClientClick=”return confirmCheckIn(this);”/>
[/html]
[javascript]
var _confirm = false;
function confirmCheckIn(button) {
if (_confirm == false) {
jQuery(‘<div>’)
.html(“<p>Do you want to check in?</p>”)
.dialog({
autoOpen: true,
modal: true,
title: “Check in?”,
buttons: {
“No”: function () {
jQuery(this).dialog(“close”);
},
“Yes”: function () {
jQuery(this).dialog(“close”);
_confirm = true;
button.click();
}
},
close: function () {
jQuery(this).remove();
}
});
}
return _confirm;
}
[/javascript]
What’s happening:
this
is passed intoconfirmCheckIn()
fromOnClientClick
, so that we have the button object to work with later- A variable
_confirm
is created and is initially set tofalse
- The dialog is displayed since
_confirm
is false false
gets returned and prevents the back-end click handler from running
When “Yes” is clicked:
- The dialog is closed,
_confirm
is set totrue
, and aclick()
is triggered on the button causingconfirmCheckIn()
to run again - This time,
_confirm
is true so a new dialog does not get shown andtrue
gets returned so the back-end handler can run
When “No” or “close” is clicked:
- The dialog simply closes