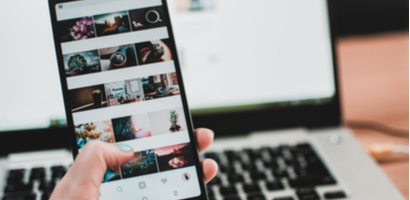
•
IE8 Memory Leaks
One of our clients wanted us to create a media gallery webpart for them. The webpart allows you to page through a folder structure/gallery of photos and videos.
Our webpart would create an image tag and then set the source each time a new image was to be displayed. This is pretty basic and it’s what you would expect. However, in IE8 this creates a memory leak, and the only way to release that memory was to close the browser. For some of the machines we tested on, after 30 images the browser would fail to load.
The DOM node types that leak in IE8 are form, button, input, select, textarea, a, img, and object.
So how do you make a media gallery webpart without image tags? After many different attempts to avoid the leak, css background-image property was the only thing that didn’t trigger it. However there was one issue, IE8 does not support re-sizing of background images.
The Fix:
As I mentioned earlier we cannot use image tags anymore as they leak. So instead we used a div tag.
[javascript]
var new_img = jQuery(‘
<div>’).css({
‘background-size’: ‘100% 100%’,
‘background-repeat’: ‘no-repeat’,
‘width’: img_width + ‘px’,
‘height’: img_height + ‘px’
})
[/javascript]
We then had to check if we were using IE8. If we are using IE8, we need to use this special filter property to be able to re-size a background image:
[javascript]
var IEBrowser =
{
Version: function()
{
var version = 999;
if (navigator.appVersion.indexOf(“MSIE”) != -1)
version = parseFloat(navigator.appVersion.split(“MSIE”)[1]);
return version;
}
}
if(IEBrowser.Version()<9)
{
new_img.css({ ‘filter’: “progid:DXImageTransform.Microsoft.AlphaImageLoader(src=’” + cache_url + “‘,sizingMethod=’scale’)” })
}
else
{
new_img.css({ ‘background-image’: ‘url(“‘ + cache_url + ‘”)’ })
}
[/javascript]
And now we have a sudo image tag that avoids the leak!